Why To Choose Python ? Benefits Of Pyhton For Competative Coder.
- Use print statements: One of the simplest ways to debug your code is by using print statements. You can print the values of variables, inputs, and outputs to see where the code is going wrong. For example:
def add_two_numbers(a, b):
print(a, b)
return a + b
result = add_two_numbers(2, "3")
print(result)
The output of this code will be:
2 3
TypeError: unsupported operand type(s) for +: 'int' and 'str'
This error message tells us that we are trying to add an integer and a string, which is not supported.
- Use the debugger: Python has an inbuilt debugger called pdb. You can use it to step through your code line by line and see what’s happening at each step. Here’s an example:
import pdb
def add_two_numbers(a, b):
pdb.set_trace()
return a + b
result = add_two_numbers(2, "3")
print(result)
When you run this code, it will pause at the line with pdb.set_trace()
. You can then step through the code using commands like n
for next, s
for step into, and c
for continue.
- Check your assumptions: Sometimes, the problem might not be with your code but with your assumptions. Make sure that you understand the problem statement correctly and that you are using the right data types and formats. For example, if the input is supposed to be a list of integers, make sure that you’re not accidentally passing a string.
- Use the built-in assert statement: You can use the assert statement to check whether a certain condition is true. If the condition is false, the program will raise an AssertionError. For example:
def add_two_numbers(a, b):
assert isinstance(a, int) and isinstance(b, int), "Inputs must be integers"
return a + b
result = add_two_numbers(2, "3")
print(result)
This will raise an AssertionError with the message “Inputs must be integers”.
- Break the code down into smaller parts: If you’re having trouble figuring out where the problem is, try breaking the code down into smaller parts and testing each part separately. This can help you isolate the problem and make it easier to fix.
These are some of the tips and tricks that you can use to debug your code on LeetCode and CodeChef. Remember, debugging is a skill that takes practice, so keep at it!
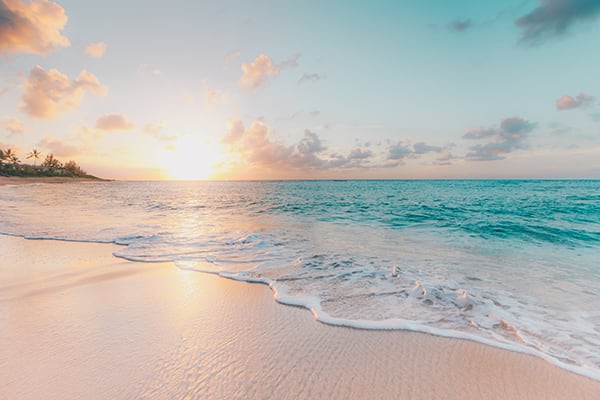
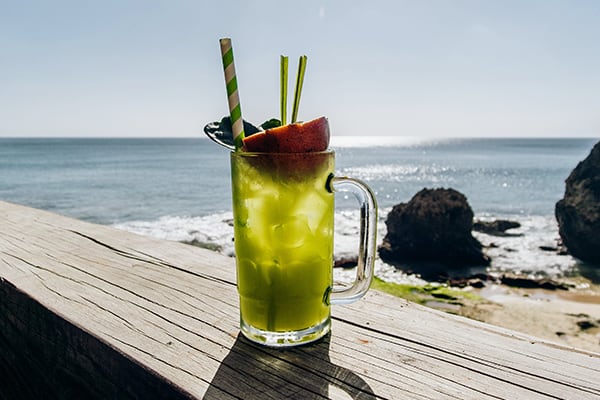
Your H3 headline can go here
Suscipit taciti primis tempor sagittis euismod libero facilisi aptent elementum felis blandit cursus gravida sociis eleifend lectus nullam dapibus netus feugiat. Curae fringilla porttitor quam sollicitudin iaculis aptent leo ligula euismod dictumst penatibus mauris eros etiam praesent volutpat posuere. Metus fringilla ullamcorper odio aliquam lacinia conubia mauris tempor etiam ultricies proin quisque lectus sociis tristique integer phasellus inceptos taciti cras pretium enim adipiscing praesent lobortis morbi cras magna vivamus.
One’s destination is never a place, but a new way of seeing things. – Henry Miller
Pretium lorem primis senectus habitasse lectus scelerisque donec ultricies tortor adipiscing fusce morbi volutpat pellentesque consectetur risus molestie curae malesuada. Dignissim lacus convallis massa mauris enim mattis magnis senectus montes mollis taciti phasellus accumsan bibendum semper blandit suspendisse faucibus nibh metus lobortis morbi cras magna vivamus per risus fermentum. Dapibus imperdiet praesent magnis ridiculus congue gravida curabitur sagittis enim magna netus inceptos iaculis sodales parturient.